

In this blog post, I will take you through how to use Python programming language to control the UR e-Series cobot. In all the below examples, we have used UR3e and the motion/path planning are based on UR3e. If you have a different version, then it’s recommended that you verify the given waypoints before executing the commands.
The latest python version can be downloaded from the official link. We can use any text editor, such as notepad++ or sublime text. In the following examples we will be using Sublime Text.
Prerequisites:
Host computer (PC or Laptop)
Python 3.X installed on Host computer and understanding of python programming
Ethernet cable connected from UR controller to Host computer
And of course, a Universal Robots cobot
Once python and the necessary text editor are installed in the host computer, we need to setup the network settings on the Teach pendant of UR and the computer.
First, connect the ethernet cable between the UR controller and the host computer. Now, navigate to Settings through the burger icon on the top right corner, then go to Network settings. In this case the cobot is set with the below IP address; remember to make sure the subnet mask is the same for both the cobot and the host computer!
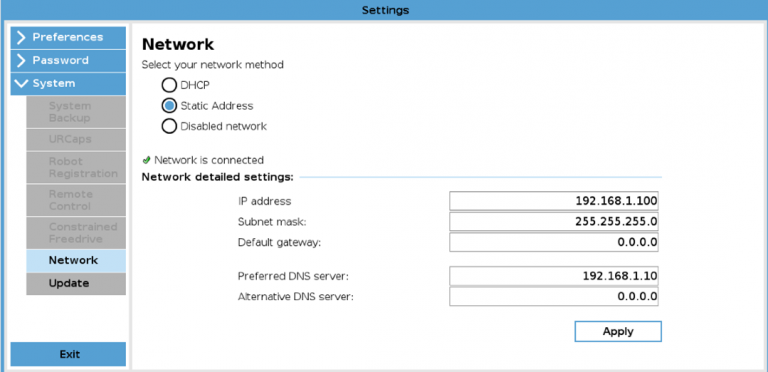
Once the network on the Host computer and UR cobot is setup, you should see the green tick with a ”Network is connected” status as shown above.
Now, on the top right corner of the teach pendant, you should see an icon with “Local” which defines that the teach pendant has full control. Once you click on “Local”, there should be a dropdown menu. Select Remote control – now your cobot is connected to your Host computer!

Up next comes the fun part – programming on python!
To start, we need to import the necessary library packages. Socket is the first package – it is for creating the connection between the computer and the Universal robot. The second package is time, which is used for adding delays between each process.
import socket
import time
Next, we need to define the “Host”, which in this case is the cobot. The “host” is the IP address of the cobot which we defined in the UR Network settings previously. The “Port” is the open port on the UR which listens for the commands sent from the computer. The required port for sending the information is “30002”.
HOST = “192.168.1.100”
PORT = 30002
Then, the code to define the socket and make the connections are as below:
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((HOST, PORT))
The “s.send” command sends the cobot script code, and can move waypoints, Digital/Analog I/Os or functions which gets executed at the robot side. Here’s an example:
s.send((“set_digital_out(0,True)”+”\n”).encode(‘utf8’))
The “\n” is the newline which has to be added after the script code, because the UR needs a newline after each command sent. In this case, for socket communication, the strings being sent need to be encoded in “utf8” which is character encoding. Python 3 does not support Unicode() function and all strings by default are unicode.
The socket connection has to be closed after the command is sent with s.close() command.

Now that your python code is ready, make sure you save the file with “.py” extension and note the location or path where the file has been saved. The sample codes are in a zip file which can be used as reference (contact us to obtain the .zip file).
To run the python file, we need to open the command line. You can go to Windows Start Menu and type “cmd” or “Win logo”+ “r” to open run command and type “cmd” and hit enter.

Now, you need to navigate to the path where you saved the python file. On the command line, type “cd”, then type the path or copy and paste the location. This can be done from sublime. Right-click on the code where you saved and click ”copy file path”.
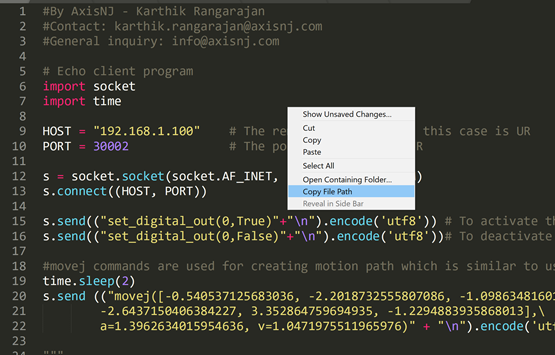
It should look something like this shown below:

When you hit enter, that should execute the commands. You should be able to see the change in the I/O tabs on the teach pendant. Check to see if the commands got executed and if there are no waypoints or motion in the part of the code.
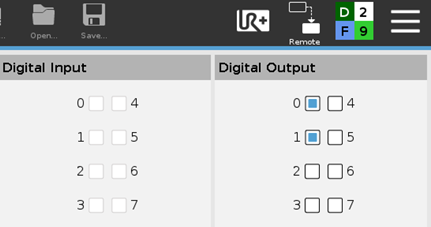
We can send the “.script” files through the socket as well.
For anyone who is not sure what these files are, in simple terms, the cobot generates a “.script” file when the “.urp” file is executed while programming on Teach pendant (in Local mode). This file can be copied and sent to the UR, which is similar to running the “.urp” file.
To test, you can create some Moves with waypoints on the teach pendant and run the program after saving. You can browse to the folder where the file is saved, and you can see that there are 3 files related to the same program/recipe:
.urp file (Universal Robot Program)
.txt file (text file of the URP)
.script file, which is generated while running the .urp file that is understood by the robot. The script file is URScript programming which is based on python. For more information, check here based on what series you have.
Now you can use this script file to the computer connected with the socket connection. With the lines of code below, you can define the path of the script file in the open function. Make sure the path to the script file is correctly directed in the line below:
f = open (“/URScript/test.script”, “rb”)
l = f.read(1024) while (l):
s.send(l)
l = f.read(1024)
We can use this method to send some of the functions, or to specifically control the grippers which are designed with URcaps. The URcap-based grippers cannot be controlled from I/O ports, so this is an easy way to control from your host computer without having to create programs on the teach pendant.
In this example, we will see the widely used Robotiq 2F gripper. In our zip file, there is compiled version of the Robotiq gripper script which includes all the functions of the gripper.
Pictured below is a list of gripper commands:
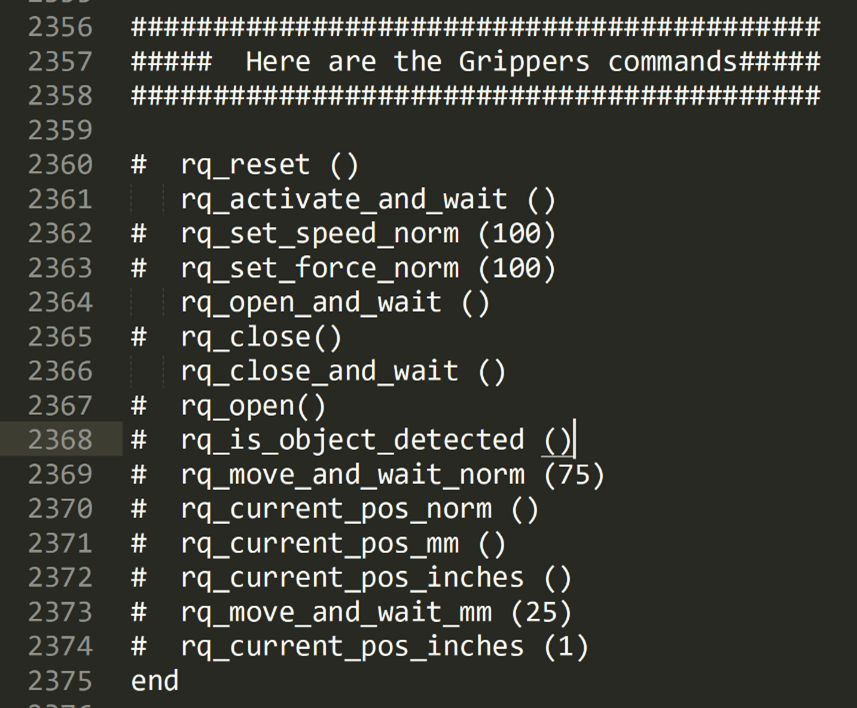
From the above list, if you remove the hashtag (#)/uncommenting the code line, that specific line gets executed. In this example, we will uncomment the functions rq_activate_and_wait () and rq_close_and_wait () which activates and actuates the gripper.
Follow the same steps as the previous process and use the gripper.script file in the open command.
Here is an example:
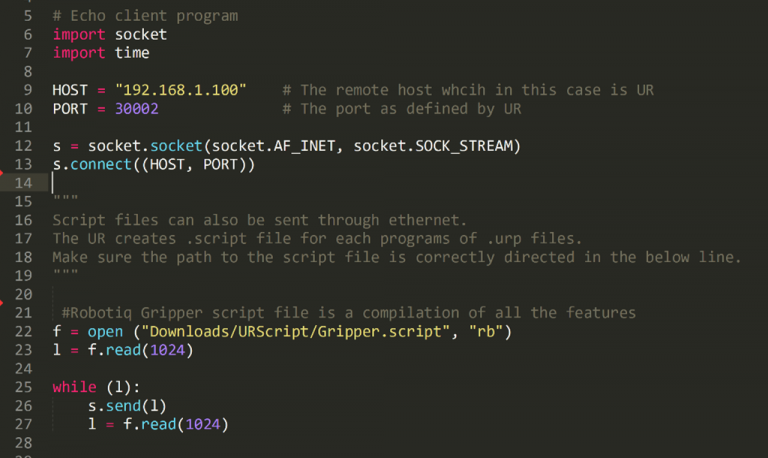
Proceeding with the same process in the previous step on the command line, executing this python file would activate and actuate the gripper. This process can be done in the same way as on GUI, meaning multiple waypoints can be defined and the gripper can be actuated.
Programming on python will provide more flexibility and allows complex programming. The applications are limitless while using python.
If you have any questions or would like to obtain the previously mentioned .zip file, please let us know!
Contact us, and we can assist you if you have any application in mind that you would like to apply this to.
Karthik Rangarajan
INDUSTRIAL AUTOMATION APPLICATION ENGINEER
Comments